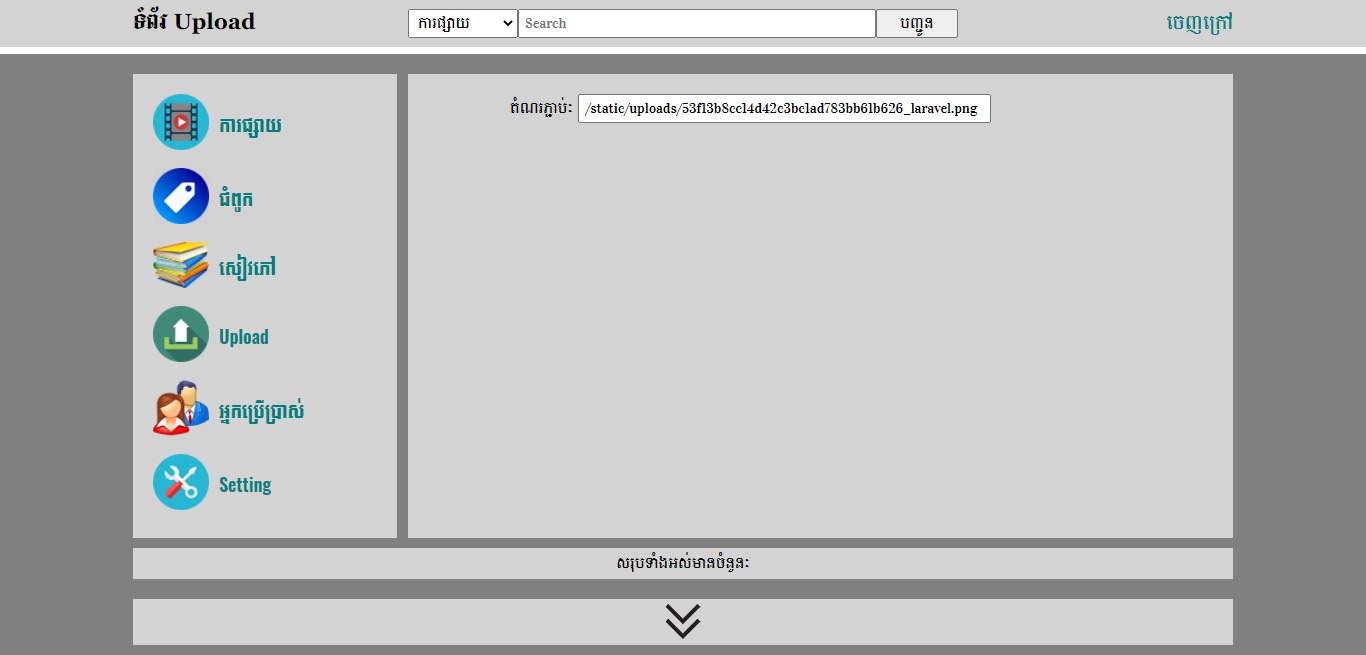
#routes/upload.py
from bottle import Bottle, redirect
from controllers.login import checkLogged
import bottle
bottle.BaseRequest.MEMFILE_MAX = 1024 * 1024
app = Bottle()
@app.route('/')
def index():
if checkLogged.call():
from controllers.dashboard.upload import get
return get.call()
else:
redirect('/')
@app.route('/', method='post')
def index():
if checkLogged.call():
from controllers.dashboard.upload import create
return create.call()
else:
redirect('/')
#controllers/dashboard/upload/create.py
import uuid, os, config
from bottle import request, template
from copy import deepcopy
def call():
kdict = deepcopy(config.kdict)
kdict['siteLogo'] = 'ទំព័រ Upload'
kdict['route'] = 'upload'
kdict['uploaded'] = 'uploaded'
upload = request.files.get('upload')
name, ext = os.path.splitext(upload.filename)
if ext not in ('.png','.jpg','.jpeg'):
return 'File extension not allowed.'
id = uuid.uuid4().hex
upload.filename = id + '_' + name + ext
kdict['url'] = '/static/uploads/' + upload.filename
save_path = 'asset/uploads'
upload.save(save_path)
return template('dashboard/index.tpl', data=kdict)
<!--views/dashboard/upload.tpl-->
<link href='/static/styles/category.css' rel='stylesheet' />
<section class='Category'>
%if 'uploaded' in data:
<form action='/dashboard/upload' method='post' enctype="multipart/form-data">
<a>តំណរភ្ជាប់ៈ</a>
<input type='text' name="link" value='{{data["url"]}}' required />
<script>
$("input[type='text']").on("click", function () {
$(this).select();
});
</script>
</form>
%else:
<form action='/dashboard/upload' method='post' enctype="multipart/form-data">
<a></a><input type='file' name="upload" required />
<a></a><input type='submit' value='Upload' />
</form>
%end
</section>
GitHub: https://github.com/Sokhavuth/REST-API
Vercel: https://rest-api-zeta.vercel.app