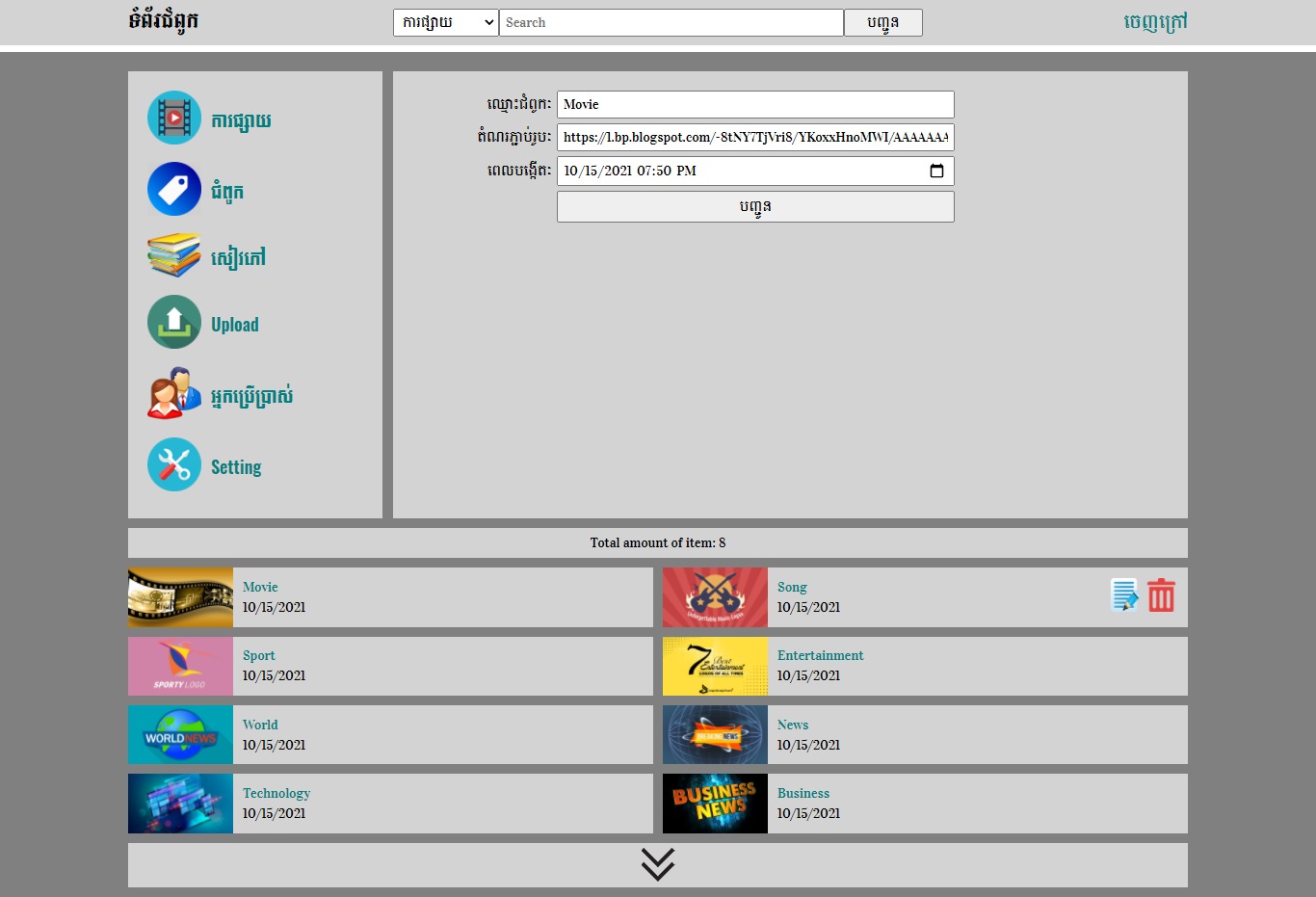
#routes/dashboard.py
import config
from bottle import Bottle, redirect
from controllers.login import checkLogged
import bottle
bottle.BaseRequest.MEMFILE_MAX = 1024 * 1024
app = Bottle()
@app.route('/')
def index():
if checkLogged.call():
from controllers.dashboard import index
return index.call()
else:
redirect('/')
@app.route('/logout')
def logout():
from controllers.dashboard import logout
logout.call()
from routes import category
app.mount('/category', category.app)
#routes/category.py
import config
from bottle import Bottle, redirect
from controllers.login import checkLogged
import bottle
bottle.BaseRequest.MEMFILE_MAX = 1024 * 1024
app = Bottle()
@app.route('/')
def index():
if checkLogged.call():
from controllers.dashboard.category import get
return get.call()
else:
redirect('/')
@app.route('/', method='post')
def create():
if checkLogged.call():
from controllers.dashboard.category import create
return create.call()
else:
redirect('/')
@app.route('/edit/<id>')
def edit(id):
if checkLogged.call():
from controllers.dashboard.category import edit
return edit.call(id)
else:
redirect('/')
#controllers/dashboard/category/edit.py
import config
from bottle import template
from copy import deepcopy
from models.categorydb import getdb, editdb
def call(id):
kdict = deepcopy(config.kdict)
kdict['siteLogo'] = 'ទំព័រជំពូក'
kdict['route'] = 'category'
kdict['edit'] = 'edit'
categories, count = getdb.call(kdict['maxItemList'])
item = editdb.call(id)
kdict['items'] = categories
kdict['count'] = count
kdict['item'] = item
return template('dashboard/index.tpl', data=kdict)
#models/categorydb/editdb.py
import setConnection
def call(id):
cursor, connection = setConnection.call()
cursor.execute("SELECT * FROM category WHERE id = ?", (id,))
item = cursor.fetchone()
cursor.close()
return item
<!--views/dashboard/category-->
<link href='/static/styles/category.css' rel='stylesheet' />
<section class='Category'>
%if 'edit' in data:
<form action='/dashboard/category' method='post'>
<a>ឈ្មោះជំពូកៈ</a><input type='text' value="{{data['item'][0]}}" name="name" required />
<a>តំណរភ្ជាប់រូបៈ</a><input type='text' value="{{data['item'][1]}}" name='link' required />
<a>ពេលបង្កើតៈ</a><input type='datetime-local' value="{{data['item'][2]}}" name='datetime' required />
<a></a><input type='submit' value='បញ្ជូន' />
<a></a><input type='hidden' value="{{data['item'][3]}}" name="editid" />
</form>
%else:
<form action='/dashboard/category' method='post'>
<a>ឈ្មោះជំពូកៈ</a><input type='text' name="name" required />
<a>តំណរភ្ជាប់រូបៈ</a><input type='text' name='link' required />
<a>ពេលបង្កើតៈ</a><input type='datetime-local' name='datetime' required />
<a></a><input type='submit' value='បញ្ជូន' />
</form>
%end
</section>
#controllers/dashboard/category/create.py
import config, uuid
from bottle import request, redirect
from models.categorydb import createdb
def call():
name = request.forms.getunicode('name')
link = request.forms.getunicode('link')
datetime = request.forms.getunicode('datetime')
edit = request.forms.getunicode('editid')
if not edit:
id = uuid.uuid4().hex
else:
id = edit
createdb.call(name, link, datetime, id, edit)
return redirect('/dashboard/category')
#models/categorydb/createdb.py
import setConnection
def call(name, link, datetime, id, edit):
cursor, connection = setConnection.call()
if not edit:
cursor.execute("INSERT INTO category VALUES(?, ?, ?, ?)", (name, link, datetime, id))
else:
sql = """UPDATE category SET
name = ?,
link = ?,
datetime = ?
WHERE id = ? """
cursor.execute(sql, (name, link, datetime, id))
connection.commit()
cursor.close()
GitHub: https://github.com/Sokhavuth/REST-API
Vercel: https://rest-api-zeta.vercel.app