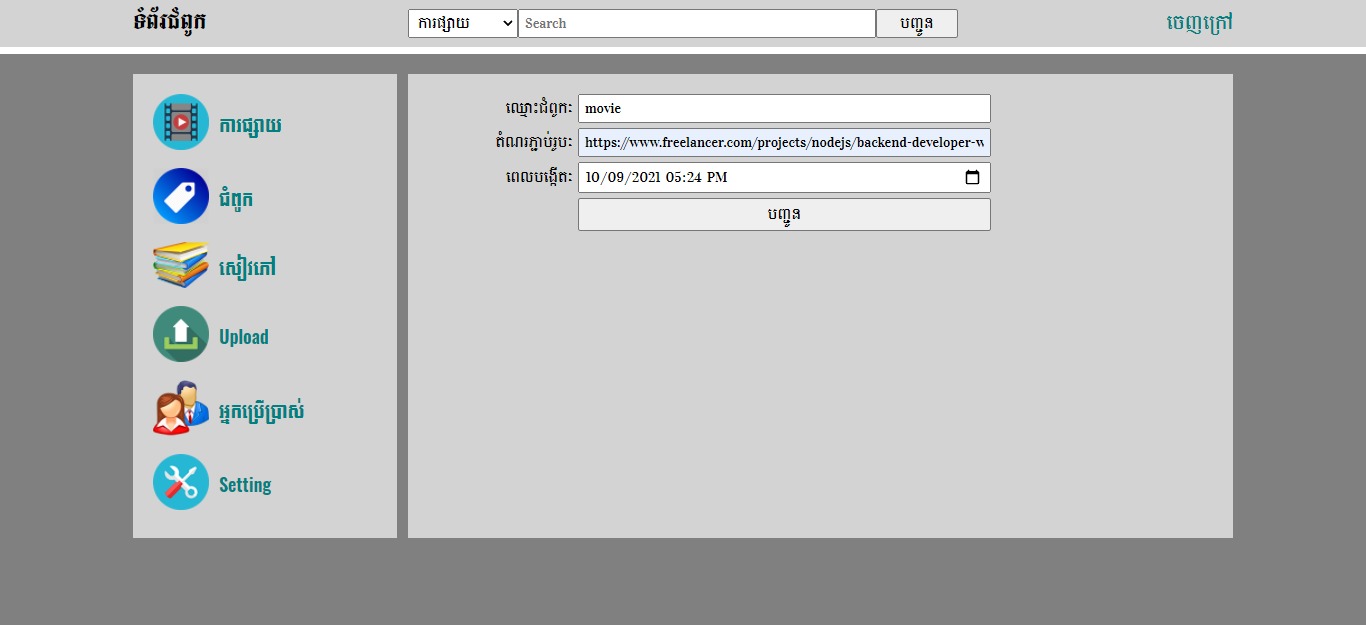
#routes/dashboard.py
import config
from copy import deepcopy
from bottle import Bottle, redirect, template, request
from controllers.login import checkLogged
import bottle
bottle.BaseRequest.MEMFILE_MAX = 1024 * 1024
app = Bottle()
@app.route('/')
def index():
if checkLogged.call():
from controllers.dashboard import index
return index.call()
else:
redirect('/')
@app.route('/logout')
def logout():
from controllers.dashboard import logout
logout.call()
@app.route('/category')
def category():
if checkLogged.call():
from controllers.dashboard.category import get
return get.call()
else:
redirect('/')
@app.route('/category', method='post')
def category():
if checkLogged.call():
from controllers.dashboard.category import create
return create.call()
else:
redirect('/')
#controllers/dashboard/category/create.py
import config
from bottle import request
from models.categorydb import createdb
def call():
name = request.forms.getunicode('name')
link = request.forms.getunicode('link')
datetime = request.forms.getunicode('datetime')
createdb.call(name, link, datetime)
return redirect('dashboard/category')
#models/categorydb/createdb.py
import setConnection
def call(name, link, datetime):
cursor, connection = setConnection.call()
cursor.execute("INSERT INTO category VALUES(?, ?, ?)", (name, link, datetime))
connection.commit()
cursor.close()
GitHub: https://github.com/Sokhavuth/REST-API
Vercel: https://rest-api-zeta.vercel.app